Binary Search Algorithm
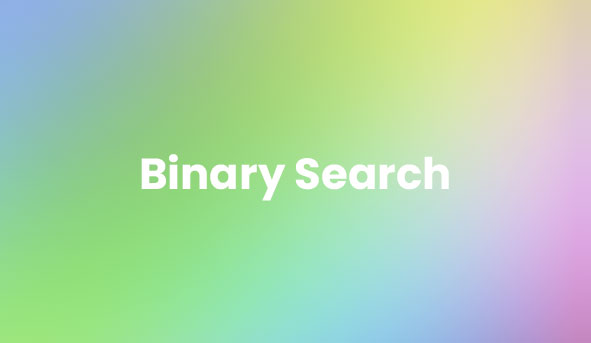
JavaScript is a programming language that is commonly used in web development. It is a client-side scripting language, which means that it is executed on the client's web browser rather than on the server.
Here is a basic tutorial on how to use JavaScript:
Setting up a basic HTML page with JavaScript First, create an HTML page with a script element that will contain your JavaScript code. The script element should be placed within the head or body of the HTML page, depending on when you want the script to be executed.
<!DOCTYPE html>
<html>
<head>
<script>
// Your JavaScript code goes here
</script>
</head>
<body>
<!-- Your HTML content goes here -->
</body>
</html>
You can also include a JavaScript file in your HTML page using the src attribute of the script element. For example:
<script src="myscript.js"></script>
The script element can also be used to include JavaScript code from an external source. For example:
<script src="https://code.jquery.com/jquery-3.4.1.min.js"></script>
Writing JavaScript code
Outputting to the console
You can use the console.log() function to output a value to the console. For example:
console.log("Hello World");
Writing comments
You can use the // or /* */ syntax to write comments in JavaScript. For example:
// This is a single-line comment
/*
This is a
multi-line comment
*/
Declaring variables
In JavaScript, you can declare variables using the var keyword. For example:
var x; // Declares a variable x
var y = 10; // Declares a variable y and assigns it a value of 10
You can also use the let and const keywords to declare variables in JavaScript. The let keyword is similar to var, but the variable is only accessible within the block it is defined in. The const keyword is used to declare a constant, which means that the value cannot be reassigned.
Data types
JavaScript has several data types, including:
- Number: a numeric value (e.g. 10, 3.14)
- String: a sequence of characters (e.g. "Hello World")
- Boolean: a value that can be either true or false
- Object: a collection of key-value pairs
- Array: a list of values
- null: a null value
- undefined: a value that has not been assigned
You can use the typeof operator to determine the data type of a value. For example:
- console.log(typeof 10); // Outputs "number"
- console.log(typeof "hello"); // Outputs "string"
- console.log(typeof true); // Outputs "boolean"
Operators
JavaScript has various operators that can be used to perform operations on values. Here are some examples:
Arithmetic operators: +, -, _, /, % (modulus) Comparison operators: ==, !=, >, <, >=, <= Logical operators: && (and), || (or), ! (not) Assignment operators: =, +=, -=, _=, /=, %= String operators: + (concatenation)
Control structures
JavaScript has several control structures that allow you to control the flow of your code.
if statements
if statements are used to execute a block of code if a certain condition is met. For example:
if (x > y) {
console.log("x is greater than y");
}
You can also include an else clause to execute a different block of code if the condition is not met:
if (x > y) {
console.log("x is greater than y");
} else {
console.log("x is less than or equal to y");
}
Loops
Loops are used to execute a block of code multiple times. JavaScript has two types of loops: for loops and while loops.
For loops
for
loops are used to execute a block of code a specified number of times. The syntax for a for loop is:
for (initialization; condition; increment) {
// Code to be executed
}
The initialization statement is executed before the loop starts. The condition is checked before each iteration of the loop, and if it is true, the loop will execute. The increment statement is executed after each iteration of the loop. Here is an example of a for loop:
for (var i = 0; i < 10; i++) {
console.log(i); // Outputs the values 0 through 9
}
While loops
while loops are used to execute a block of code as long as a certain condition is true. The syntax for a while loop is:
while (condition) {
// Code to be executed
}
Here is an example of a while loop:
var i = 0;
while (i < 10) {
console.log(i); // Outputs the values 0 through 9
i++;
}
Functions
Functions are blocks of code that can be called from elsewhere in your code. You can define a function using the function keyword, followed by the function name, a list of parameters in parentheses, and the code to be executed in curly braces. For example:
function sayHello(name) {
console.log("Hello, " + name + "!");
}
sayHello("John"); // Outputs "Hello, John!"
You can also define functions using arrow syntax, like this:
const sayHello = (name) => {
console.log("Hello, " + name + "!");
};
sayHello("Jane"); // Outputs "Hello, Jane!"
Objects
Objects are collections of key-value pairs that can be used to store data in JavaScript. You can create an object using curly braces and specifying the key-value pairs within the braces. For example:
const person = {
name: "John",
age: 30,
occupation: "programmer",
};
You can access the values within an object using dot notation or bracket notation. For example:
console.log(person.name); // Outputs "John"
console.log(person["age"]); // Outputs 30
You can also add or modify properties of an object using dot notation or bracket notation:
person.location = "New York";
person["status"] = "single";
Arrays
You can access the values in an array using their index, which is the position of the value in the array. The first value in the array has an index of 0, the second value has an index of 1, and so on. You can access a value in an array using square brackets and the index. For example:
console.log(numbers[0]); // Outputs 1
console.log(words[2]); // Outputs "three"
You can also use the length property of an array to determine the number of elements in the array:
console.log(numbers.length); // Outputs 5
You can use the push() method to add a new value to the end of an array:
numbers.push(6);
You can use the pop() method to remove the last value from an array:
numbers.pop();
You can also use the shift() method to remove the first value from an array, and the unshift() method to add a new value to the beginning of an array.
Events
JavaScript allows you to attach functions to events that occur on a webpage. For example, you can use the onclick attribute to specify a function to be executed when an element is clicked. Here is an example:
<button onclick="sayHello('John')">Click me</button>
You can also use the addEventListener() method to attach an event listener to an element:
const button = document.getElementById("myButton");
button.addEventListener("click", sayHello);
Conclusion
This is just a basic overview of some of the features of JavaScript. There is much more to learn, including advanced concepts such as asynchronous programming, prototypal inheritance, and working with the DOM. To learn more about JavaScript, we recommend reading documentation and tutorials online, or taking an online course.